In this article we will be sharing how we can update email templates via upgrade scripts.
We have already shared some of our knowledge about running upgrade scripts and how helpful they are in automating database changes in deployment processes. I would recommend checking our first article "Create or update blocks and pages via Upgrade Scripts" for more details on how to run upgrade scripts. I will only explain the lines of code for updating email templates via scripts in here.
To add new transactional email template in Admin, you go to:
- Admin > System > Transactional Emails
- Add New Template
- Load the default template + Click Load Template
- This will load up Content of the default Magento template for each template you want to style
- All default email templates are located in: app/locale/en_US/template/email/
- Transactional emails are inside
sales/
folder - Header and Footer inside
html/
folder
- Transactional emails are inside
- Add a new Template Name
- Update the email content in Template Content field.
The same can be also done through upgrade script
In this example we are updating New Order Email and loading its Magento default template:
<?php
$installer = $this;
$installer->startSetup();
$newOrderEmail = Mage::getModel('core/email_template');
$newOrderEmail->setData('template_code', 'New Order Email');
$newOrderEmail->setData('template_type', Mage_Core_Model_Email_Template::TYPE_HTML);
$newOrderEmail->setData('template_subject', ': New Order # ');
$newOrderEmail->setData('orig_template_code', 'sales_email_order_template');
$newOrderEmail->setData('orig_template_variables', 'add email variables here');
$newOrderEmailContent = <<<'END_OF_EMAIL'
here goes email content..
END_OF_EMAIL;
$newOrderEmail->setData('template_text', $newOrderEmailContent);
$newOrderEmail->save();
$installer->endSetup();
?>
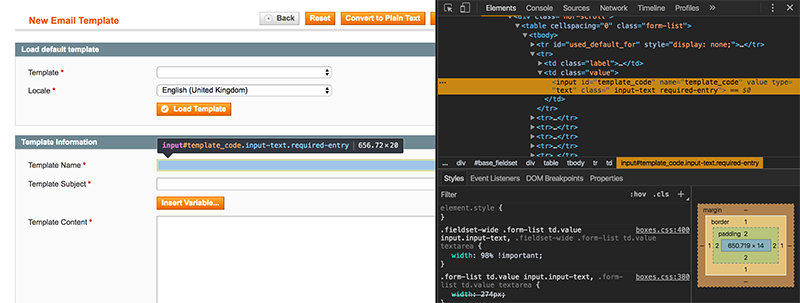
which matches with template_code
field in our database. This is where our data is stored:
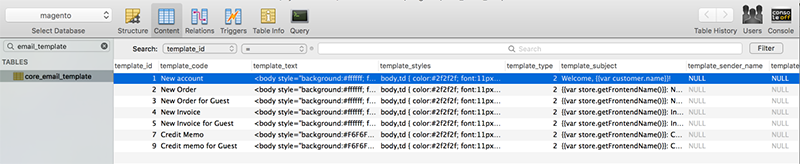
Update existing Transaction Email template via upgrade script
<?php
$installer = $this;
$installer->startSetup();
$newOrderEmail = Mage::getModel('core/email_template');
$newOrderEmail->loadByCode('New Order Email');
$newOrderEmailContent = <<<'END_OF_EMAIL'
here goes email content..
END_OF_EMAIL;
$newOrderEmail->setData('template_text', $newOrderEmailContent);
$newOrderEmail->save();
$installer->endSetup();
?>
This works very similar as the first example, but this time we call our already existing template with $newOrderEmail->loadByCode('New Order Email');
targeting Template Name New Order Email field (template_code
field in the database).
Changing settings in Admin to use your new Email Header/Footer Template:
- Go to System > Configuration > Design > Transactional Emails
- Choose the newly created email template from Email Header Template / Email Footer Template dropdown
Changing it through upgrade script:
<?php
Mage::getConfig()->saveConfig('design/email/header', $emailHeader->getId(), 'default', 0);
?>
Our $emailHeader
variable has already been set when we created our new Email Header Template.
Changing settings in Admin to use your new Transactional email templates:
- Go to System > Configuration > SALES > Sales Emails
- Most of the transactional email templates can be updated in this section (Order, Invoice, Shipment etc..)
Changing it through upgrade script:
<?php
Mage::getConfig()->saveConfig('sales_email/order/template', $newOrderEmail->getId(), 'default', 0);
?>
Our $newOrderEmail
variable has already been set when we created our new Order Email Template.
Changing settings in Admin to use your new Back to Stock email template:
- Go to System > Configuration > CATALOG > Catalog > Product Alerts
- Update your new template in Stock Alert Email Template dropdown
Changing it through upgrade script:
<?php
$installer->setConfigData('catalog/productalert/email_stock_template', $backToStockEmail->getId());
?>
Our $backToStockEmail
variable has already been set when we created our new Back to Stock Email Template.
That’s about it! I hope you find upgrade scripts useful as we do and we are always happy to receive any thoughts at @meanbee.